Introduction
If you work in data science, software engineering, or any related field, you may have come across the term JSON. If you are just coming into these fields, you might be confused.
JSON stands for JavaScript Object Notation. As the word “notation” might imply, JSON is a way to represent data independent of the platform. For example, it is like a PDF (which is the same across different platforms such as mobile, desktop and web) for data. Simply put, JSON is a file format that is used to store and exchange data.
JSON was born in 2002 (defined in the ECMA-404 standard) and has become widely popular thanks to its ease of use and flexibility. Although it originated from the world of JavaScript, it quickly spread other programming languages.
JSON strings are usually stored in .json files and transmitted over the network with an application/json MIME type.
An alternative to JSON is XML (Extensible Markup Language), but JSON is better in many ways. While both are human-readable and machine-readable, JSON is much easier to read and faster for computers to process. Furthermore, JSON is processed (or parsed) using a JavaScript parser (which is included in most web-browsers) while XML requires a separate XML parser. This is where the “JavaScript” in “JSON” comes into play.
How to JSON
The syntax of JSON is very similar to JavaScript, so it would be familiar if you have previous experience with JavaScript.
In JSON, data is stored in a set of key-value pairs. Each data element contains a key with which you can modify, add, or delete the data element.
Here’s an example of a JSON string:
{
"firstName": "foo",
"lastName": "bar",
"age": 82
}
From this snippet you can see that the keys are encapsulated in double quotes (“firstName”, “lastName”), a colon separates the key and the value, and the value can be of different types (“foo”, “bar”, 82).
Sets of key-value pairs are separated by a comma.
Note: All keys must be strings, which means they must be enclosed in double quotes. Also, there is a comma after every key-value pair except for the last pair, indicating that a new element is being registered.
Spacing (spaces, tabs, new lines) does not matter in a JSON file. The above is equivalent to:
{"firstName": "foo","lastName":"bar","age":82}
or
{"firstName":
"foo", "lastName":
"bar","age":
82}
but well-formatted data is better to understand.
Data Types
JSON supports the below basic data types:
- number: any number that is not wrapped in quotes
- string: any set of characters wrapped in quotes
- boolean: true or false
- array: a list of values, wrapped in square brackets
- object: a set of key-value pairs, wrapped in curly brackets
- null: the null word, which represents an empty value
Any other data type must be serialized to a string (and then deserialized) to be stored in JSON.
Encoding and Decoding JSON in Javascript
ECMAScript 5 in 2009 introduced the JSON object in the JavaScript standard, which provides amount other things the JSON.parse() and JSON.stringify() methods.
Before it can be used in a JavaScript program, JSON must be parsed in string format and converted into data that JavaScript can use.
JSON.parse() takes a JSON string as a parameter, and returns an object that contains the parsed JSON:
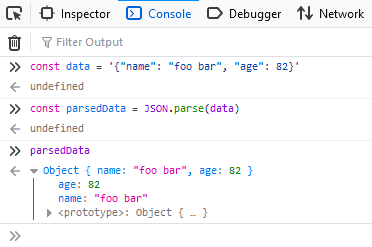
JSON.stringify() takes a JavaScript object as its parameter, and returns a string representing it in JSON:
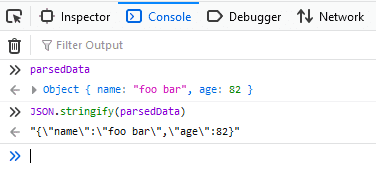
JSON.parse() can also accept an optional second argument called the reviver function. You can use this to hook into the parsing and perform any custom operation:
JSON.parse(string, (key, value) => {
if (key === 'name') {
return `Name: ${value}`
} else {
return value
}
})
Looking for how to encode and decode JSON in python? Check out our article here: Python JSON: Encoding and Decoding
Nesting Objects
In JSON, a value can also be an object (which is also a key-value pair). This object is like another JSON file. It is enclosed with curly braces and contains key-value pairs, except that it is inside our original JSON file instead of having its own file.
You can organize the data in a JSON file with a nested object like this:
{
"name": {
"firstName": "foo",
"lastName": "bar"
},
"age": 82,
"country": {
"details": {
"name": "Foobarica"
}
}
}
Nesting Arrays
JSON provides a data type for efficient storage of a list of data called an array. It is an ordered set of items which can be of any data type.
Let’s add a list of friends to the above example:
{
"name": {
"firstName": "foo",
"lastName": "bar"
},
"age": 82,
"country": {
"details": {
"name": "Foobarica"
}
},
“friends”: [
“bar foo”,
“foo foo”,
“bar bar”
]
}
The array is enclosed with square brackets, and each element is a newline followed by a comma except for the last one. The new line is not necessary, but it helps the ease of reading the code for humans.
Online Tools for JSON
There are many useful tools that you can use to help you with writing JSON.
One of them is JSONLint, a JSON Validator. With it you can check if a JSON string is valid.
JSONFormatter is a great tool for formatting a JSON string so that it is more readable according to your conventions.
Conclusion
We hope this article helped you get familiar with JSON. In this article, we covered what JSON is, why it is useful, and finally, how to do (some) JSON. We also covered the different JSON data types (string, number, array, object, and boolean).
If you any questions or thoughts on the tutorial, feel free to reach out in the comments below.